Note
Go to the end to download the full example code. or to run this example in your browser via Binder
Load and explore pose tracks#
Load and explore an example dataset of pose tracks.
Imports#
from movement import sample_data
from movement.io import load_poses
from movement.plots import plot_centroid_trajectory
Define the file path#
This should be a file output by one of our supported pose estimation frameworks (e.g., DeepLabCut, SLEAP), containing predicted pose tracks. For example, the path could be something like:
# uncomment and edit the following line to point to your own local file
# file_path = "/path/to/my/data.h5"
For the sake of this example, we will use the path to one of
the sample datasets provided with movement
.
file_path = sample_data.fetch_dataset_paths(
"SLEAP_three-mice_Aeon_proofread.analysis.h5"
)["poses"]
print(file_path)
/home/runner/.movement/data/poses/SLEAP_three-mice_Aeon_proofread.analysis.h5
Load the data into movement#
ds = load_poses.from_sleap_file(file_path, fps=50)
print(ds)
<xarray.Dataset> Size: 27kB
Dimensions: (time: 601, space: 2, keypoints: 1, individuals: 3)
Coordinates:
* time (time) float64 5kB 0.0 0.02 0.04 0.06 ... 11.96 11.98 12.0
* space (space) <U1 8B 'x' 'y'
* keypoints (keypoints) <U8 32B 'centroid'
* individuals (individuals) <U10 120B 'AEON3B_NTP' 'AEON3B_TP1' 'AEON3B_TP2'
Data variables:
position (time, space, keypoints, individuals) float32 14kB 770.3 ......
confidence (time, keypoints, individuals) float32 7kB nan nan ... nan nan
Attributes:
source_software: SLEAP
ds_type: poses
fps: 50.0
time_unit: seconds
source_file: /home/runner/.movement/data/poses/SLEAP_three-mice_Aeon...
The loaded dataset contains two data variables:
position
and confidence
.
To get the position data:
Select and plot data with xarray#
You can use the sel
method to index into xarray
objects.
For example, we can get a DataArray
containing only data
for a single keypoint of the first individual:
da = position.sel(individuals="AEON3B_NTP", keypoints="centroid")
print(da)
<xarray.DataArray 'position' (time: 601, space: 2)> Size: 5kB
770.3 1.062e+03 773.3 1.062e+03 773.3 ... 618.6 194.6 618.4 194.6 616.4
Coordinates:
* time (time) float64 5kB 0.0 0.02 0.04 0.06 ... 11.96 11.98 12.0
* space (space) <U1 8B 'x' 'y'
keypoints <U8 32B 'centroid'
individuals <U10 40B 'AEON3B_NTP'
We could plot the x, y coordinates of this keypoint over time,
using xarray
’s built-in plotting methods:
da.plot.line(x="time", row="space", aspect=2, size=2.5)
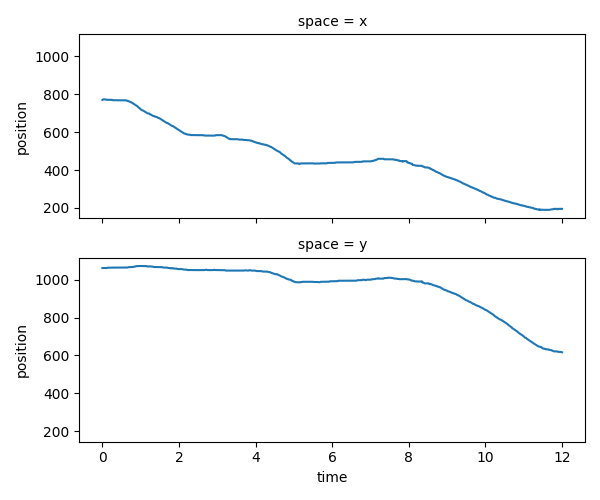
<xarray.plot.facetgrid.FacetGrid object at 0x7fbe1e23c140>
Similarly we could plot the same keypoint’s x, y coordinates for all individuals:
da = position.sel(keypoints="centroid")
da.plot.line(x="time", row="individuals", aspect=2, size=2.5)
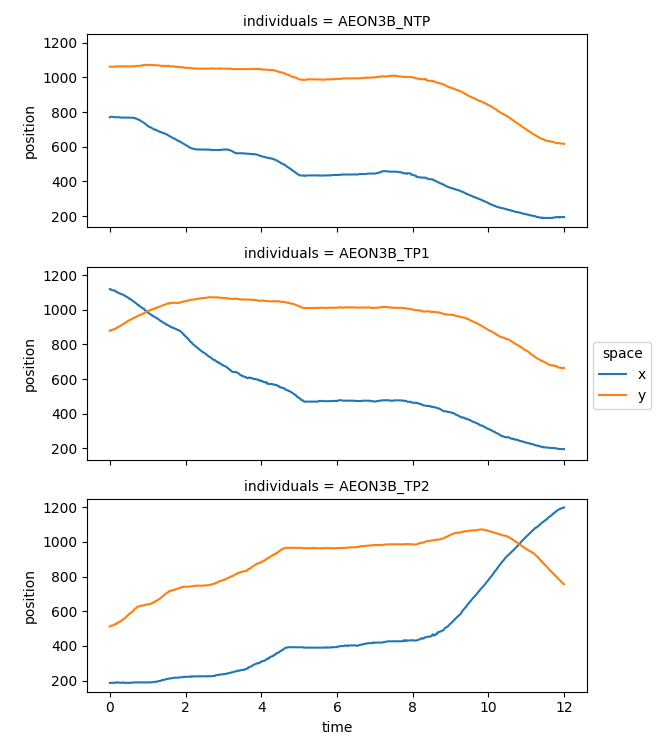
<xarray.plot.facetgrid.FacetGrid object at 0x7fbe1e25b6e0>
Trajectory plots#
We are not limited to xarray
’s built-in plots.
The movement.plots
module provides some additional
visualisations, like plot_centroid_trajectory()
.
mouse_name = "AEON3B_TP1"
fig, ax = plot_centroid_trajectory(position, individual=mouse_name)
fig.show()
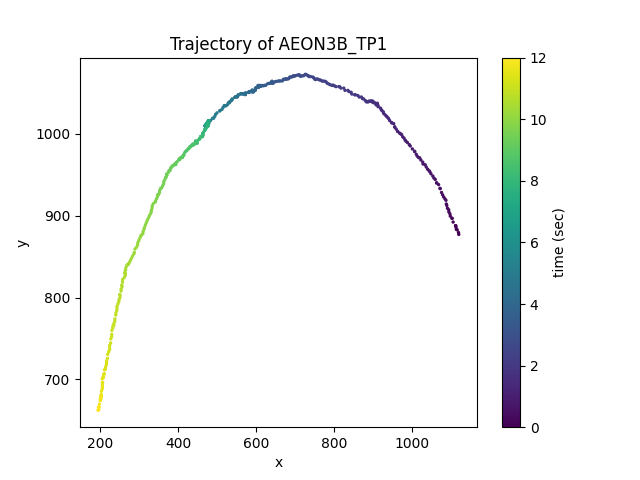
Total running time of the script: (0 minutes 0.718 seconds)